Collection Framework Library - Notes By ShariqSP
Frameworks in Java
In Java programming, a framework is a reusable, pre-written set of libraries, classes, and components that provide a structured approach to developing applications. Frameworks simplify the development process by offering predefined templates, guidelines, and functionalities for common tasks, allowing developers to focus on application-specific logic rather than low-level details.
Why Do We Need Frameworks?
Frameworks serve several purposes in Java development:
- Productivity: Frameworks provide ready-to-use components and functionalities, enabling developers to build applications faster and more efficiently.
- Consistency: Frameworks enforce consistent coding practices and architectural patterns, ensuring that applications adhere to best practices and standards.
- Scalability: Frameworks often incorporate scalability features such as modularity, dependency injection, and built-in support for distributed computing, making it easier to scale applications as they grow.
- Maintenance: By promoting code reuse, encapsulation, and separation of concerns, frameworks simplify maintenance and updates, reducing the risk of bugs and technical debt.
- Community Support: Many frameworks have active communities of developers who contribute plugins, extensions, and documentation, providing valuable resources and support for developers.
Popular Java Frameworks:
There are numerous frameworks available in the Java ecosystem for various purposes, including web development, enterprise applications, testing, and more. Some popular Java frameworks include:
- COllection Framework Library
- Spring Framework
- Hibernate
- Apache Struts
- JavaServer Faces (JSF)
- Apache Maven
- Apache Kafka
- JUnit
- Play Framework
- Vert.x
- Quarkus
Collection Framework in Java
The Collection Framework in Java provides a unified architecture for representing and manipulating collections of objects. It is an essential part of Java programming and is extensively used in various applications. Students will learn the following key aspects of the Collection Framework:
- Understanding the hierarchy of collection interfaces and classes
- Working with different types of collections such as lists, sets, queues, and maps
- Implementing collection interfaces and utilizing built-in collection classes
- Exploring common algorithms and operations provided by the Collection Framework
- Learning best practices for selecting and using appropriate collection types
- Understanding the performance characteristics of different collection implementations
Components of Collection Framework:
The Collection Framework includes the following key components:
- Interfaces: Define standard behaviors and contracts for different types of collections, such as lists, sets, queues, and maps.
- Implementations: Concrete classes that implement the interfaces and provide specific implementations of collection types.
- Algorithms: Utility methods for performing common operations on collections, such as sorting, searching, filtering, and iterating.
Advantages of Collection Framework:
- Reusability: Collection Framework provides reusable components that can be used across different applications and projects.
- Flexibility: The framework supports a wide range of collection types and provides interchangeable implementations, allowing developers to choose the most suitable data structure for their needs.
- Performance: Collection Framework includes efficient data structures and algorithms optimized for various operations, ensuring high performance and scalability.
- Consistency: By adhering to a common set of interfaces and conventions, the framework promotes consistency and interoperability among different parts of an application.
Usage Examples:
Here's an example demonstrating the use of Collection Framework interfaces and implementations:
import java.util.*;
public class Main {
public static void main(String[] args) {
// Create a list of strings
List list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.add("C++");
// Iterate over the list
for (String language : list) {
System.out.println(language);
}
}
}
In this example, an ArrayList is used to store a list of strings, and the elements are iterated using a for-each loop.
Hierarchy of Collection Framework
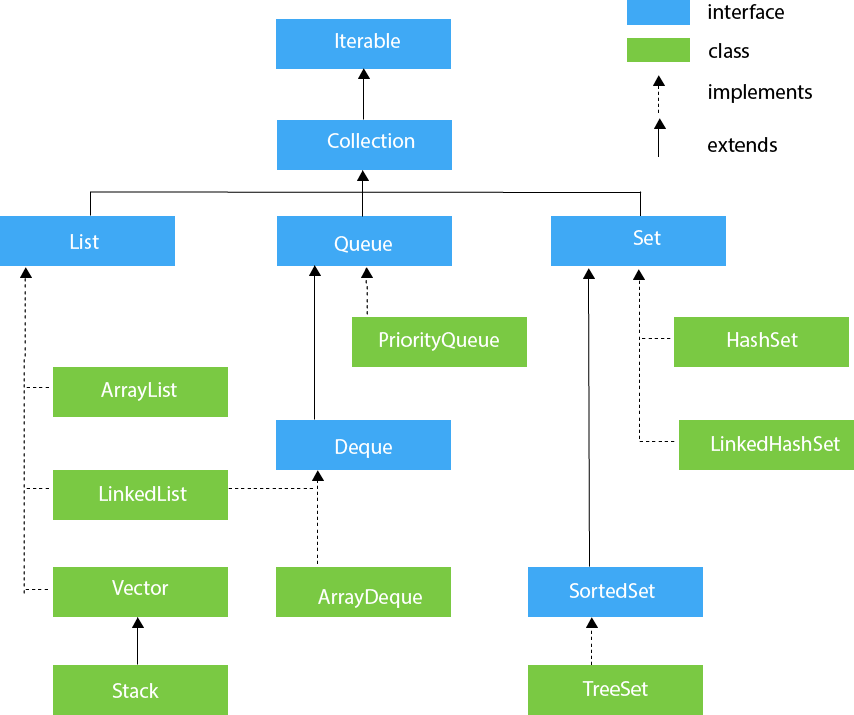
Explanation of Iterable and Collection Interfaces in Java
Iterable Interface:
The Iterable
interface is the root interface in the Java Collection Framework hierarchy. It represents a collection of objects that can be iterated over, allowing sequential access to its elements. The Iterable
interface defines a single method:
public interface Iterable<T> {
Iterator<T> iterator();
}
The iterator()
method returns an Iterator
object, which allows iterating over the elements of the collection in a sequential manner. Classes that implement the Iterable
interface can be used with the enhanced for loop (for-each loop) in Java.
Collection Interface:
The Collection
interface extends the Iterable
interface and represents a group of objects known as elements. It is a high-level interface that provides operations for basic collection manipulation, such as adding, removing, and querying elements. The Collection
interface serves as the root interface for all collection types in Java.
The Collection
interface defines several methods for common collection operations, including:
boolean add(E e)
: Adds the specified element to the collection.boolean remove(Object o)
: Removes the specified element from the collection.int size()
: Returns the number of elements in the collection.boolean contains(Object o)
: Returnstrue
if the collection contains the specified element.Iterator<E> iterator()
: Returns an iterator over the elements in the collection.- And many more...
Classes that implement the Collection
interface represent different types of collections, such as lists, sets, and queues. These classes provide concrete implementations of the Collection
interface and define specific behavior for different collection types.
The Collection Framework in Java is organized into a hierarchy of interfaces and classes that represent various types of collections and provide common behaviors and operations. Understanding this hierarchy is essential for effectively working with collections in Java.
Collection Interface:
The Collection
interface is the root interface in the Collection Framework hierarchy. It defines the basic operations that all collections support, such as adding, removing, and querying elements. Collections that implement this interface represent groups of objects, known as elements.
Subinterfaces:
- List: Ordered collection of elements that allows duplicate elements and provides positional access.
- Set: Unordered collection of unique elements.
- Queue: Collection designed for holding elements prior to processing. Supports insertion and removal operations at both ends.
- Deque: Double-ended queue that supports insertion and removal at both ends.
Map Interface:
The Map
interface represents a mapping between a key and a value. Unlike collections, maps do not implement the Collection
interface. Each key-value pair in a map is treated as an entry.
Classes:
- List Implementations: Classes that implement the
List
interface, such asArrayList
,LinkedList
, andVector
. - Set Implementations: Classes that implement the
Set
interface, such asHashSet
,LinkedHashSet
, andTreeSet
. - Queue Implementations: Classes that implement the
Queue
interface, such asPriorityQueue
. - Map Implementations: Classes that implement the
Map
interface, such asHashMap
,LinkedHashMap
, andTreeMap
.
Utilities:
The Collection Framework also provides utility classes and methods for working with collections, such as sorting, searching, and iterating.
Comparable and Comparator in Java
In Java, Comparable
and Comparator
are interfaces used for sorting objects based on their natural ordering or a custom ordering defined by the user.
Comparable Interface:
The Comparable
interface is used to define the natural ordering of objects. Classes that implement the Comparable
interface can be sorted based on their natural ordering.
Example:
Consider a class Student
that implements the Comparable
interface based on the student's ID.
public class Student implements Comparable {
private int id;
private String name;
// Constructor, getters, setters
@Override
public int compareTo(Student other) {
return Integer.compare(this.id, other.id);
}
}
In this example, the compareTo()
method compares two students based on their IDs.
Comparator Interface:
The Comparator
interface is used to define custom ordering for objects that do not implement the Comparable
interface or for overriding the natural ordering of objects.
Example:
Consider a Comparator
for comparing students based on their names.
import java.util.Comparator;
public class StudentNameComparator implements Comparator {
@Override
public int compare(Student s1, Student s2) {
return s1.getName().compareTo(s2.getName());
}
}
In this example, the compare()
method compares two students based on their names.
Comparable | Comparator |
---|---|
Interface is implemented by the class whose objects are to be sorted. | Separate class is created to implement the Comparator interface. |
Objects are sorted based on their natural ordering. | Objects can be sorted based on custom ordering defined by the comparator. |
compareTo() method is used to define the natural ordering of objects. | compare() method is used to define the custom ordering of objects. |
Implementation affects the natural ordering of the class itself. | Implementation does not affect the natural ordering of the class itself. |
Useful when the class has a natural ordering that should be preserved across all collections. | Useful when different sorting criteria are required based on different use cases. |
Using the iterator() Method in Java
The iterator()
method in Java is used to obtain an iterator over the elements of a collection. It returns an Iterator
object, which allows iterating over the elements of the collection sequentially. Iterators provide a way to access the elements of a collection without exposing its underlying implementation.
How to Use iterator() Method:
The iterator()
method is typically called on instances of classes that implement the Iterable
interface. Once obtained, the iterator can be used to traverse the elements of the collection using methods such as next()
, hasNext()
, and remove()
.
Example:
Suppose we have a list of integers and we want to iterate over its elements using the iterator()
method:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class IteratorExample {
public static void main(String[] args) {
// Create a list of integers
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
numbers.add(4);
numbers.add(5);
// Obtain an iterator over the list
Iterator<Integer> iterator = numbers.iterator();
// Iterate over the elements using the iterator
while (iterator.hasNext()) {
Integer number = iterator.next();
System.out.println(number);
}
}
}
In this example, we create an ArrayList
of integers and add some elements to it. We then obtain an iterator using the iterator()
method and use a while
loop to iterate over the elements of the list. Inside the loop, we use the next()
method to retrieve each element and hasNext()
method to check if there are more elements.
The use of iterators provides a way to traverse collections in a uniform and efficient manner, regardless of the specific implementation of the collection.
Interview Questions and MCQs on Collection Framework in Java
Interview Questions:
- What is the Java Collection Framework?
- Explain the main interfaces in the Collection framework.
- What is the difference between Collection and Collections?
- What are the key benefits of using the Collection framework?
- What is the difference between ArrayList and LinkedList?
- How does the HashMap work internally?
- What is the purpose of the Comparable interface?
- What is the role of the Comparator interface?
- Explain the difference between HashSet and TreeSet.
- What are the advantages of using ConcurrentHashMap over Hashtable?
- What is the significance of the Iterator interface?
- How do you synchronize a collection in Java?
- What is the difference between fail-fast and fail-safe iterators?
- Explain the purpose of the ListIterator interface.
- How do you create an immutable collection in Java?
- What is the purpose of the Queue interface?
- Explain the difference between poll() and remove() methods in Queue.
- What is the role of the Deque interface?
- How does the LinkedHashMap maintain insertion order?
- What are the advantages of using LinkedList over ArrayList in certain scenarios?
Multiple Choice Questions (MCQs):
- Which interface is the root interface of the Java Collection Framework?
a) Collection
b) List
c) Set
d) Map
Answer: a) Collection - Which collection class allows duplicate elements?
a) HashSet
b) TreeSet
c) LinkedHashSet
d) None of the above
Answer: c) LinkedHashSet - Which method is used to remove all elements from a collection?
a) removeAll()
b) clear()
c) deleteAll()
d) erase()
Answer: b) clear() - Which interface provides a way to access the elements of a collection sequentially?
a) Iterator
b) Enumeration
c) ListIterator
d) Traverser
Answer: a) Iterator - Which collection class represents a resizable array?
a) ArrayList
b) LinkedList
c) Vector
d) All of the above
Answer: d) All of the above